WordPress sites typically use PHP to serve HTML content that’s preloaded with necessary data. However, thanks to the WordPress REST API, this approach is not the only way to build WordPress sites. The REST API allows you to communicate with your site’s backend by sending and receiving JSON objects. You can use it to build powerful themes and plugins for your site that have more dynamic access to data, thus facilitating deeper levels of interactivity. You can even build decoupled frontends using libraries such as React, which then access your site’s data via the API.
In this article, you’ll learn more about the WordPress REST API and the common errors you’re likely to encounter while working with it. You’ll learn potential causes of these errors, as well as some possible solutions.
About the REST API
The WordPress REST API is not required for building a functioning WordPress site, so many developers may not be aware of it or be familiar with what it does. Normally, WordPress sites are built in PHP, including the themes and plugins. This could present an unwanted limitation to developers who would prefer to build their site using a different technology or who want to build a complementary mobile application that uses data from their site.
In these situations, you can use the REST API as a language- and framework-agnostic way of accessing all the same data you would in PHP. The API works with any language that can make HTTP requests and parse JSON responses, encompassing nearly all modern programming languages. Below is an example of the kind of data that is exposed by the REST API:
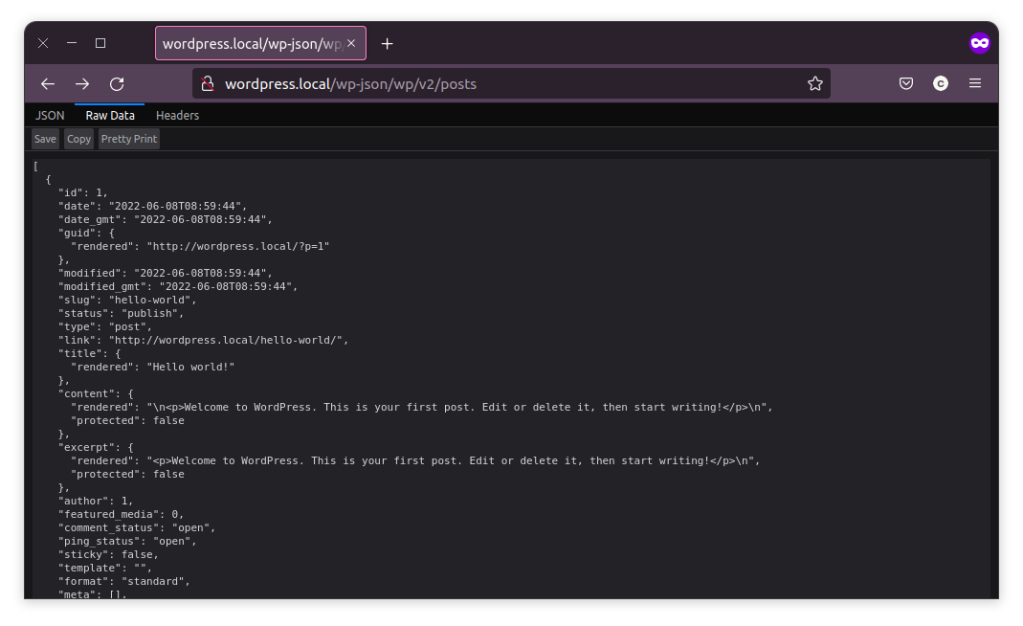
You can adopt the REST API incrementally—perhaps first using it to add interactivity to your existing WordPress site, then eventually using it to implement a fully custom JavaScript frontend. At this stage, WordPress would essentially act like a headless CMS; it would allow you to keep all of your existing content and plugins while gaining the benefits of a decoupled front end, such as greater flexibility in how you can develop your site.
If you implement the REST API, though, especially when using it on a new site for the first time, you may deal with the occasional error. Some of these errors are common, but fortunately they have simple solutions.
Common REST API Errors
The following are some of the more common errors you might encounter when using the WordPress REST API.
Bad Permalink Settings
One common cause of issues in WordPress is misconfigured permalink settings, which can come up whether or not you’re using the REST API. In this case, when you try to navigate to the REST API endpoint, you’ll be greeted with a 404 error page like this:
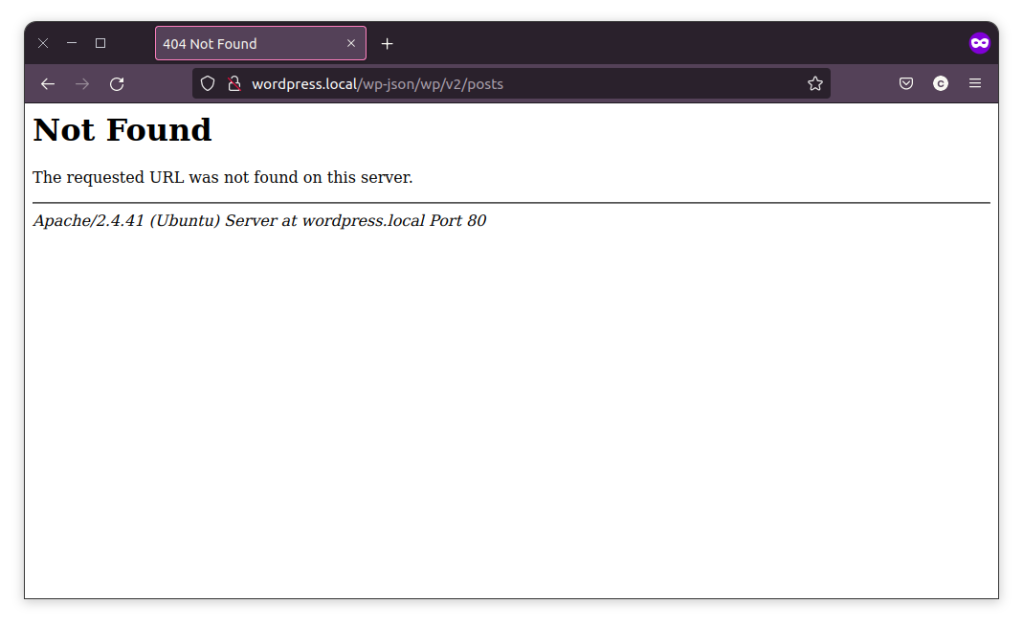
There are a few different causes for this. In this particular instance, the issue is some missing apache2
config:
# /etc/apache2/sites-enables/000-default.conf
<VirtualHost *:80>
ServerAdmin webmaster@localhost
DocumentRoot /var/www/html
ErrorLog ${APACHE_LOG_DIR}/error.log
CustomLog ${APACHE_LOG_DIR}/access.log combined
# This following block was missing, thus breaking permalinks
<Directory /var/www/html>
Options Indexes FollowSymLinks
AllowOverride All
Require all granted
</Directory>
</VirtualHost>
Another common cause of broken permalinks is having a misconfigured .htaccess
file. If you go to your WP Admin dashboard and then navigate to Settings > Permalinks, you can select your permalink style. When you do this, WordPress will attempt to update your .htaccess
file accordingly, but in cases where file system permissions prevent it from being able to do so, you will see an error like this:
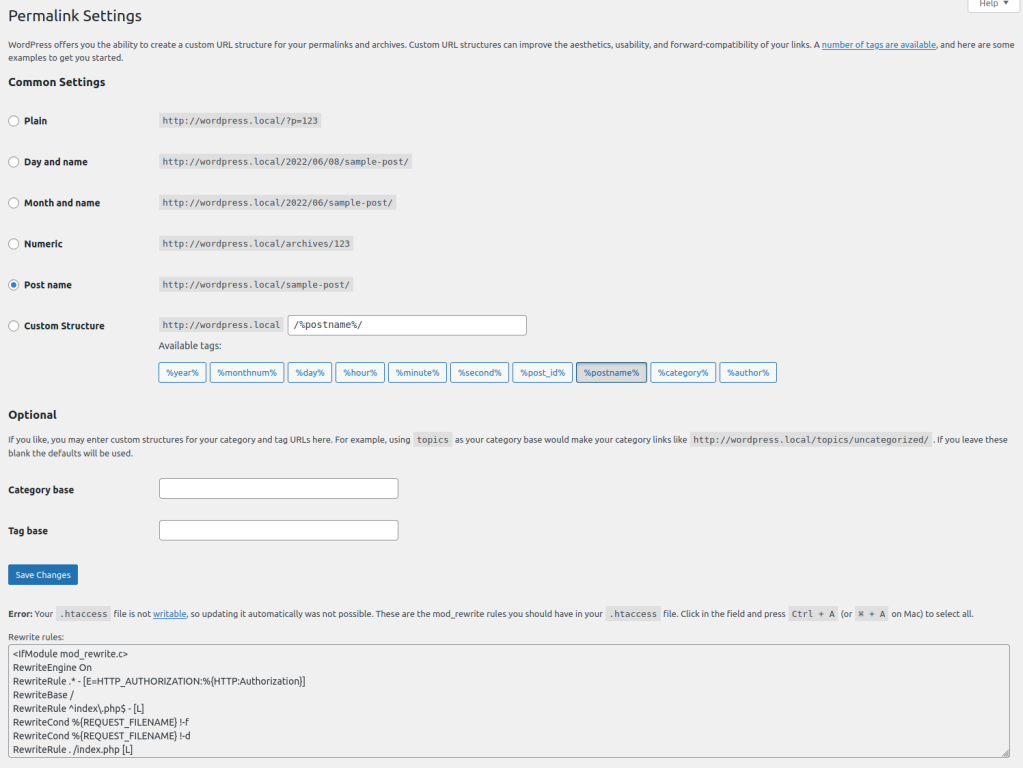
In this case, you need to either resolve your permission issues or manually update the .htaccess
file with the snippet provided by WordPress below the error. Generally speaking, it’s better to update the permission issue if you can. This typically means ensuring that your WordPress installation directory is owned by whichever user the web server is running under (often www-data
or nginx
) and that the appropriate file and directory permissions are set.
If you have shell access to your server, you can use the following command to find out which user your web server is running under:
ps aux | egrep '(apache|httpd|nginx)'
In this case, you can see that it is running under the www-data
user:
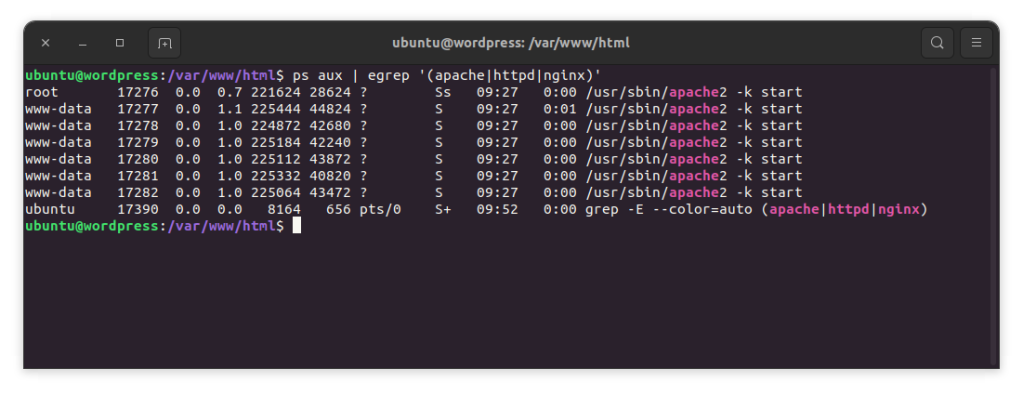
With this information, you can update the owner of your WordPress installation by navigating to the appropriate directory and running the following command:
sudo chown -R www-data-www-data .
You may also find that you need to update the actual file and directory permissions if these are currently misconfigured. For more information on doing this, check the official documentation about changing permissions.
Disabled API
The REST API may be disabled on your site. There are some legitimate reasons why you might want this. For example, by default, the API exposes the usernames of those who have posted content on your site. This helps ensure the complete gathering of information. However, it could theoretically be used to assist in a brute force attack against your admin panel, because it gives the attacker some known usernames to try. That can be enough reason for some developers to disable the REST API.
The API could be disabled by custom PHP code or a WordPress plugin that offers such functionality. There are quite a few plugins that offer this ability, generally represented as security-centric. If you suspect an errant plugin may be disabling your REST API access, check for installed plugins in that vein. Some plugins that are known to offer this functionality include:
It is also possible to disable the REST API without a plugin. For example, adding the following snippet to your wp-includes/functions.php
file will cause the REST API to cease functioning:
function disable_rest($access) {
return new WP_Error('access denied', 'REST API Disabled', ['status' => 403]);
}
add_filter('rest_authentication_errors', disable_rest);
Attempts to access the REST API would then return the following response:
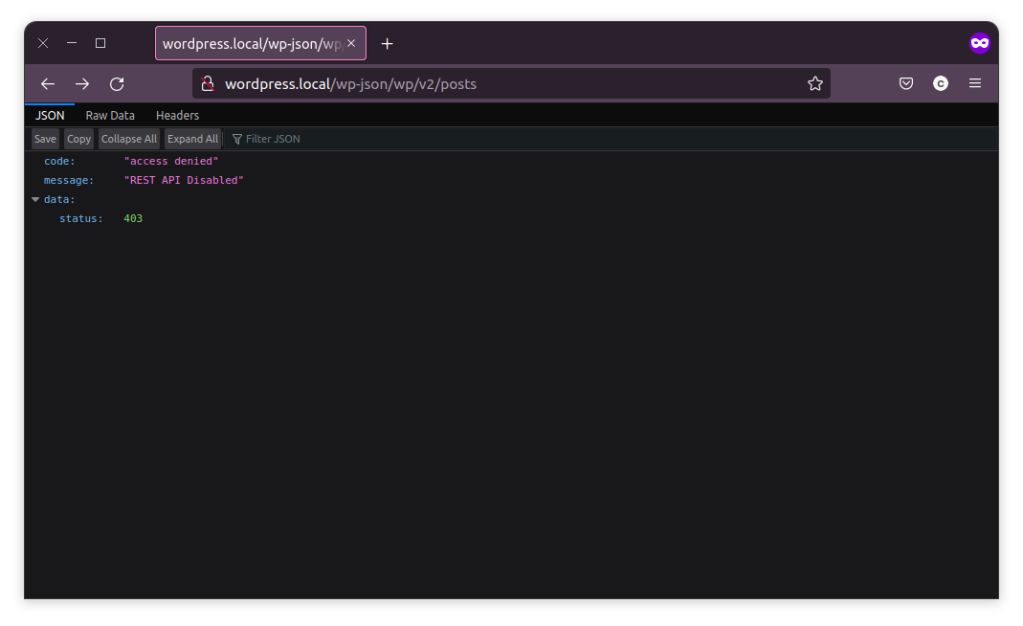
Between plugins and custom code, there are countless ways that the REST API could have been manually disabled. If you suspect this is the problem and are unsure where to look, start by disabling all of your plugins (use discretion here, as some plugins may be critical to your site’s functionality) to see if this allows access to the REST API. If it does, start re-enabling plugins until you find which ones are causing the issue.
If no plugins seem to be the cause, you can also look through any custom code that has been added to your site, such as in the functions.php
file. Performing a global search for mentions of phrases like “REST” or “API” could be a good starting point, but unfortunately, each site can be so different that if you get this far without finding the cause, you will likely need to commit to some detective work.
General Errors
Another thing to be wary of is common errors and how they can present themselves through the REST API. Ultimately, the REST API is just another interface through which you can interact with your site. This means that when there are mistakes or errors in the code, the REST API is liable to stop working as expected, just like any other code. Consider the following contrived example, in which a hook is used to change the content
of all posts to “Some new content”:
function change_post_content( $post_object ) {
// A contrived example of applying a filter
$post_object->post_content = 'Some new content' // A missing semicolon
return $post_object;
}
add_action('the_post', 'change_post_content');
As the comment points out, there is a missing semicolon. The impact of this mistake is quite severe, simply a blank page with no information to guide you toward the cause:
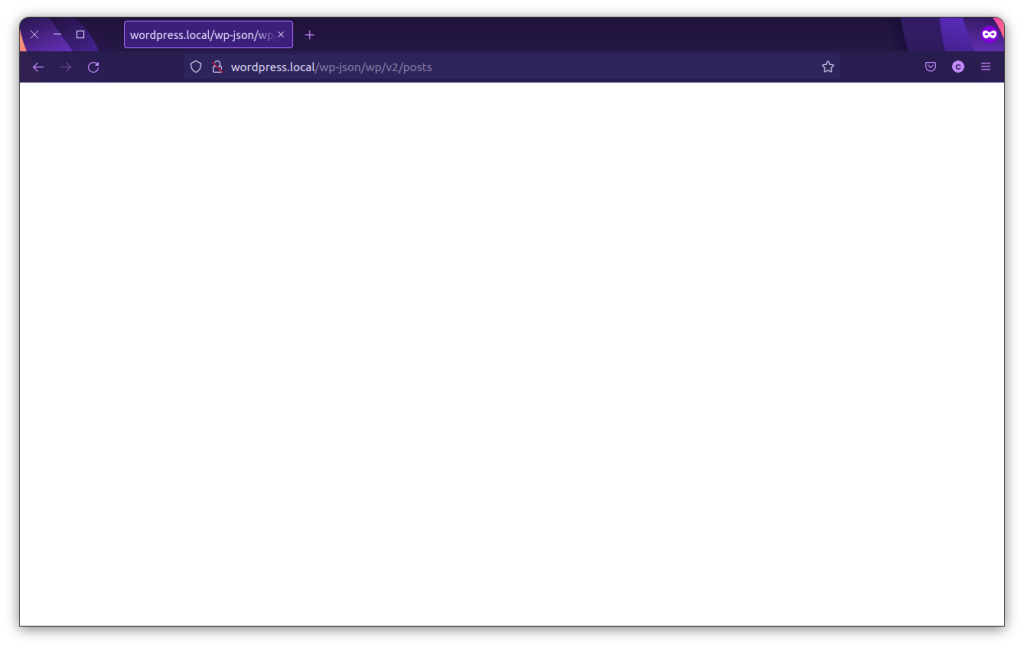
One of the reasons why you won’t get any guidance here is because exposing that kind of low-level information would be a security concern. Thankfully, if you were to check the error logs on the server, in this case found at /var/log/apache2/error.log
, you would see that the most recent error says something like:
[Sun Sep 18 10:25:10.145284 2022] [php7:error] [pid 1177] [client 192.168.1.176:44796] PHP Parse error: syntax error, unexpected 'return' (T_RETURN) in /var/www/html/wp-includes/functions.php on line 8471, referrer: http://wordpress.local/wp-json/wp/v2/posts
This is much more helpful, and quite clearly suggests where the error is.
Another thing to be mindful of is how to manage your own errors. As a developer, you will likely find yourself in a position where you need to add some error handling to a plugin, or to custom code you’re writing. The WordPress documentation has specific guidance around how you should typically deal with errors through the use of the WP_Error
class. It is essential to make sure that you don’t just throw exceptions in custom code without handling them, because doing so will typically result in a similar outcome to the previous example, though with slightly different output:
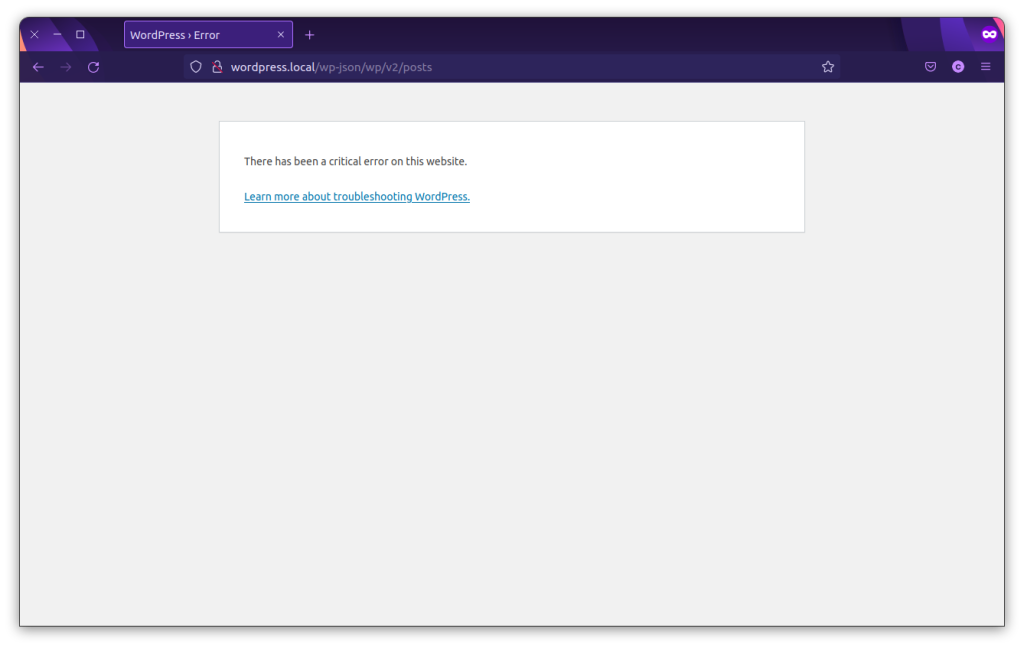
Like the previous example, if you run into something like this, the error logs are a good first place to check. In this case, the logs once again point you to exactly where you need to look:
[Sun Sep 18 10:29:54.862517 2022] [php7:error] [pid 768] [client 192.168.1.176:37230] PHP Fatal error: Uncaught Exception: Something went wrong in /var/www/html/wp-includes/functions.php:8472nStack trace:n#0
…
Conclusion
The WordPress REST API can add a lot of value to your site by allowing more dynamic ways to access your site’s data. You can use the API to add enhanced interactivity to your site, create new decoupled frontends, or build mobile applications that use your site’s data. There are some common errors that can cause difficulties with your API usage, such as improper permalink settings, incomplete rewrite configs, and security plugins or custom code that disable API access. Fortunately, there are also solutions for these errors.
It’s important to be aware of these issues as you develop your WordPress project. If you decide to use the WordPress REST API, you can easily solve these issues and prevent any inefficiencies or delays in your workflow so that you’re better able to produce high-quality results.