By default the main RSS feed of your WordPress site only includes standard posts. WordPress does create extra feeds for custom post types, but what if you wanted those posts in your main feed? In this tutorial I’ll show you the code needed to include custom post types into the main RSS feed in WordPress.
Let’s dive straight into the code. We simply need to hook into the request
filter which filters the query variables that are passed to the default main SQL query for a given page in WordPress. We’ll need to make sure we are targeting the RSS feed and that the post_type variable hasn’t already been defined.
The code to add a custom post type to your RSS feed looks like this:
/**
* Modify the RSS feed post types.
*
* @link https://www.wpexplorer.com/how-to-add-custom-post-types-to-rss-feed/
*/
add_filter( 'request', function( $query_vars ) {
if ( isset( $query_vars['feed'] ) && ! isset( $query_vars['post_type'] ) ) {
$query_vars['post_type'] = [ 'post', 'YOUR_CUSTOM_POST_TYPE_NAME' ];
}
return $query_vars;
} );
Make sure to modify where it says YOUR_CUSTOM_POST_TYPE_NAME accordingly. Also, note that what we are doing is setting the value of the post_type variable so we need to return an array of all the post types we want included.
Important RSS Caching Notice: If you add the code snippet to your site and visit your RSS feed you may not see the custom posts included. WordPress caches your RSS feed to keep it fast. In order to clear the cache you can create a new post or save any existing post.
And if you wanted to include all custom post types in your RSS feed instead of returning an array of post types you can use the core get_post_types()
function. You can see an example snippet below:
/**
* Include all custom post types in the main RSS feed.
*
* @link https://www.wpexplorer.com/how-to-add-custom-post-types-to-rss-feed/
*/
add_filter( 'request', function( $query_vars ) {
if ( isset( $query_vars['feed'] ) && ! isset( $query_vars['post_type'] ) ) {
$query_vars['post_type'] = get_post_types( [ 'public' => true ], 'names' );
}
return $query_vars;
} );
Should You Include Custom Posts in the Main RSS Feed?
Whether you should or shouldn’t include custom post types in your main RSS feed really depends on your site. On the Total Theme Documentation site all of the documentation articles are added under a “docs” custom post type. And I don’t add any standard posts to the site. So in this example the RSS feed would be empty by default. Thus, I use a little code to set the RSS feed use the docs post type.
I think in most cases you probably would keep your custom posts separate from the main feed. Posts such as portfolio, staff, testimonials and products don’t make sense added to an RSS feed. I really can’t think of many scenarios where you would need to include custom posts into the main feed.
But, if you do want to include them, it’s easy!
If you’ve decided to include a custom post type into your main RSS feed you may want to remove the post type specific feed. Personally, I use Yoast SEO on all my sites and I generally use their settings that removes all the RSS feeds excerpt the main one like such:
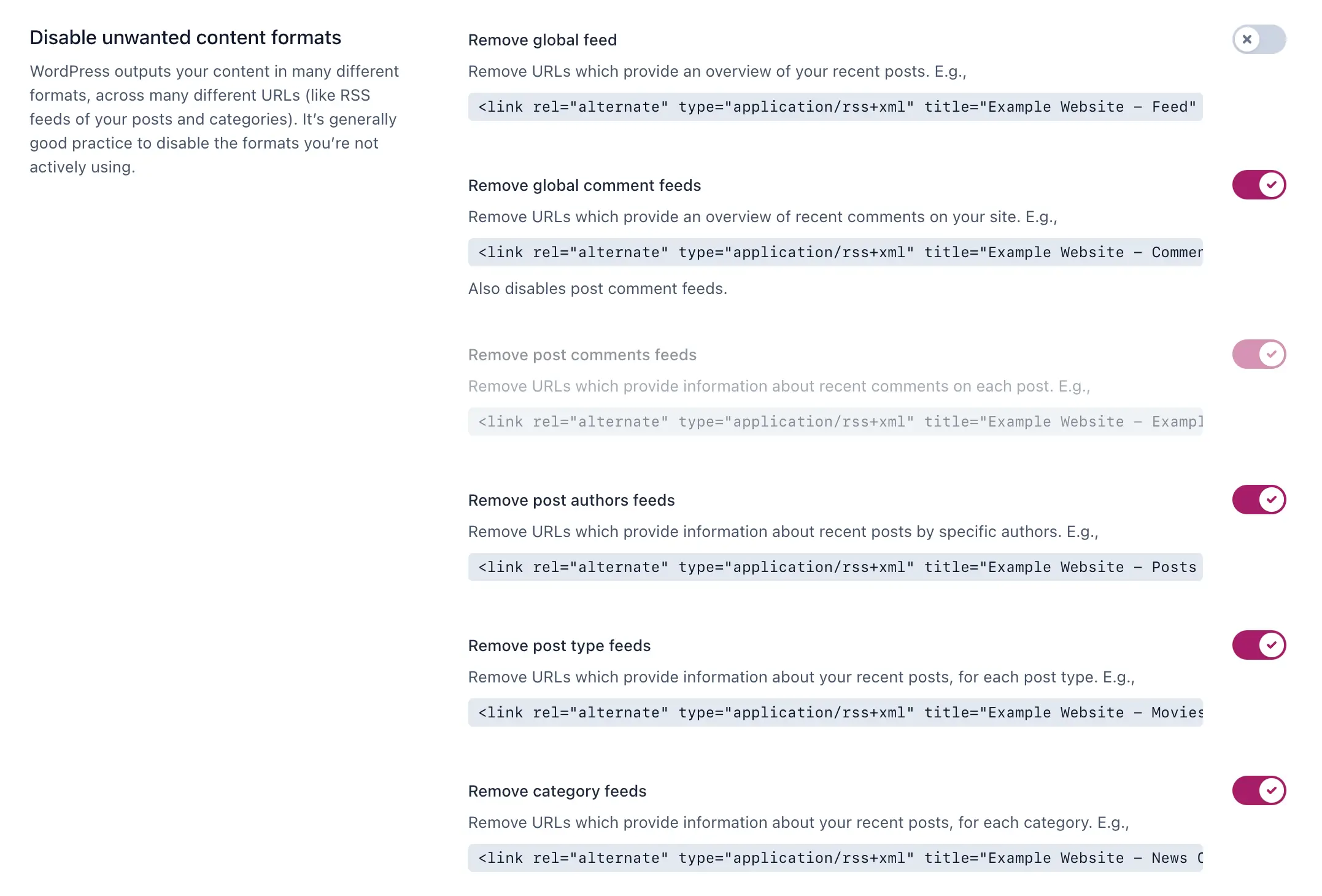
If you are looking for the code to remove a specific post type RSS feed it’s a bit complex because we need to do a couple things.
Disable Feed Permalink Structure for the Post Type
If you are registering your custom post type yourself then you can simply edit your register_post_type function arguments and set the rewrite feed argument to false. Otherwise you can hook into the register_post_type_args filter to modify the post type arguments like such:
/**
* Remove rss feed permalink structure for a custom post type.
*
* @link https://www.wpexplorer.com/how-to-add-custom-post-types-to-rss-feed/
*/
add_filter( 'register_post_type_args', function( $args, $post_type ) {
if ( 'YOUR_CUSTOM_POST_TYPE_NAME' === $post_type ) {
if ( ! isset( $args['rewrite'] ) ) {
$args['rewrite'] = [];
}
$args['rewrite']['feeds'] = false;
}
return $args;
}, 10, 2 );
By disabling the permalink structure for the post type RSS feeds will make it so if you go to your-site.com/post-type/feed/ the URL will return a 404. Make sure you go go to Settings > Permalinks and re-save the settings to flush your permalinks after adding this code.
Simply removing the feed permalink structure doesn’t actually disable the custom post type feed. WordPress will instead link to the feed using the format: your-site.com/post-type?feed=rss2. So you will want to remove these links for SEO reasons. To remove the meta tags from the site header we can use some code like this:
/**
* Remove meta links to a custom post type RSS feed.
*
* @link https://www.wpexplorer.com/how-to-add-custom-post-types-to-rss-feed/
*/
add_action( 'wp', function() {
if ( is_post_type_archive( 'YOUR_CUSTOM_POST_TYPE_NAME' ) ) {
remove_action('wp_head', 'feed_links_extra', 3 );
}
} );
This code will remove the custom post type RSS feed from the archive. Of course the alternative is to set the has_archive
argument when registering your post type to false
. Which you would generally do most of the time so that you can use a static page for your custom post type and have more control over the layout.